Chat API
The Web Widget (Classic) includes a chat component that lets users chat with an agent. The component is represented by thechat
object ofwebWidget
.
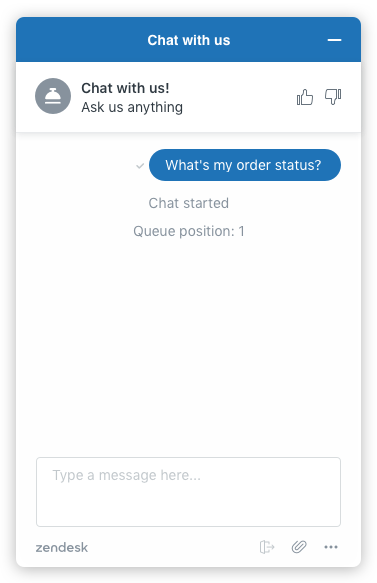
Settings
Thechat
object has the following settings:
- suppress
- title
- badge
- concierge
- connectOnPageLoad
- departments
- hideWhenOffline
- menuOptions
- navigation
- prechatForm
- profileCard
- offlineForm
- notifications
- authenticate
The integrated Chat experience is enabled inAdmin CenterunderChannels>Classic>Web Widgetand turning the Chat toggle on. If you're not a Support admin, ask one to enable it for you.
Example
<scripttype="text/javascript">
window.zESettings={
webWidget:{
chat:{
suppress:false,
notifications:{
mobile:{
disable:true
}
}
}
}
};
script>
Commands
The chat component has the following commands:
- chat:send
- get chat:isChatting
- get chat:department
- get chat:departments
- chat:end
- updatePath
- on chat:connected
- on chat:start
- on chat:end
- on chat:status
- on chat:departmentStatus
- on chat:unreadMessages
- popout
- on chat:popout
- chat:addTags
- chat:removeTags
- chat:reauthenticate
chat:send
zE('webWidget', 'chat:send', message
Makes the visitor send a message. Starts a chat session if one is not already in progress.
Parameters
message
: String. Message to be sent
Passing non-string types results in an error. No message is sent.
Example
<scripttype="text/javascript">
zE('webWidget','chat:send',"I'd like the Jambalaya, please");
script>
get chat:isChatting
zE('webWidget:get', 'chat:isChatting');
Checks whether a chat session is in progress.
Parameters
None
Return value
Boolean
get chat:department
zE('webWidget:get', 'chat:department', department
Returns an object containing information about the specified department, including itsid
,name
, andstatus
. Otherwise returnsundefined
if the department is not found or not enabled.
Note: This function should only be called after the widget is connected. See the examples.
Parameters
department
: Integer or string. Id or name of the department
Any other input types will returnundefined
.
Examples
<scripttype="text/javascript">
zE('webWidget:on','chat:connected',function(){
constdepartment=zE('webWidget:get','chat:department','Accounting');
console.log(department);
});
script>
<scripttype="text/javascript">
zE('webWidget:on','chat:connected',function(){
constdepartment=zE('webWidget:get','chat:department',5);
console.log(department);
});
script>
Return value
- An object containing information about the specified department, including its id, name, and status
- Otherwise
undefined
if the department is not found or not enabled
get chat:departments
zE('webWidget:get', 'chat:departments');
返回一个列表的所有部门containi启用ng information about each department including itsid
,name
, andstatus
. Returnsundefined
if chat is not connected.
Note: This function should only be called after the widget is connected. See the example.
Parameters
None
Return value
- An array of objects containing information about each department, including its
id
,name
, andstatus
.
Example
<scripttype="text/javascript">
zE('webWidget:on','chat:connected',function(){
constdepartments=zE('webWidget:get','chat:departments');
departments.forEach(department=>{
console.log(department);
});
});
script>
chat:end
zE('webWidget', 'chat:end');
Ends the current chat session.
Parameters
None
updatePath
zE('webWidget', 'updatePath', options
Programmatically updates the visitor’s web path.
Note: Chat triggers set to run "when a visitor has loaded the chat widget" will be fired when the visitor path is changed.
Parameters
options
: Object (optional). An object with two keys:url
(for the URL of the page) andtitle
(to set the page's title). If not specified or invalid, the location and title of the current page will be used.
If passed non-object types or objects with other keys, the chat reverts back to using the location and title of the current page.
Examples
<scripttype="text/javascript">
// Without options
zE('webWidget','updatePath');
script>
<scripttype="text/javascript">
// With options
zE('webWidget','updatePath',{
url:'http://example.com',
title:"Ready to rock'n'roll!"
});
script>
on chat:connected
zE('webWidget:on', 'chat:connected', callback
Registers a callback to be fired when the widget successfully connects to the server.
Parameters
callback
:函数。The callback to perform on chat connection
Example
<scripttype="text/javascript">
zE('webWidget:on','chat:connected',function(){
console.log('successfully connected to Zendesk Chat!');
});
script>
on chat:start
zE('webWidget:on', 'chat:start', callback
Registers a callback to be fired when a chat starts.
Parameters
callback
:函数。回调执行聊天开始
Example
<scripttype="text/javascript">
zE('webWidget:on','chat:start',function(){
console.log('successfully started a Zendesk Chat!');
});
script>
on chat:end
zE('webWidget:on', 'chat:end', callback
Registers a callback to be fired when a chat ends.
A chat only ends when the visitor (and not the agent) ends the chat, or when the visitor has been idle for an extended period of time.
Parameters
callback
:函数。The callback to perform on chat end
Example
<scripttype="text/javascript">
zE('webWidget:on','chat:end',function(){
console.log('successfully ended a Zendesk Chat session!');
});
script>
on chat:status
zE('webWidget:on', 'chat:status', function(status
Registers a callback to be fired when the account status changes.
Parameters
callback
:函数。The callback to perform on account status change. Contains one parameter,status
, a string that can be one of 'online'|'away'|'offline'
Example
<scripttype="text/javascript">
zE('webWidget:on','chat:status',function(status){
console.log('This chat session is now',status);
});
script>
on chat:departmentStatus
zE('webWidget:on', 'chat:departmentStatus', function(department
Registers a callback to be fired when a department status changes.
Parameters
callback
:函数。The callback to perform on each department status change. Contains one parameter,department
, an object that contains thename
,id
andstatus
of the changed department.
Example
<scripttype="text/javascript">
zE('webWidget:on','chat:departmentStatus',function(dept){
console.log('department',dept.name,'changed to',dept.status);
});
script>
on chat:unreadMessages
zE('webWidget:on', 'chat:unreadMessages', function(number
Registers a callback to be fired when the number of unread messages changes.
Parameters
callback
:函数。The callback to perform on unread messages. Contains one parameter,number
, an integer detailing the number of unread messages.
Example
<scripttype="text/javascript">
zE('webWidget:on','chat:unreadMessages',function(number){
console.log(`It seems you have${number}unread messages!`);
});
script>
popout
zE('webWidget', 'popout');
Attempts to open the live chat widget in a new window on desktop. The popout command functions when the Chat status is "online". It may not work on some devices or configurations.
Important: This command should only be called from a user event listener callback. See example.
Parameters
None
Example
<ahref="javascript:void(zE('webWidget','popout'))">Open chat in new windowa>
on chat:popout
zE('webWidget:on', 'chat:popout', callback
Registers a callback to be performed when a chat pop-out window is opened.
Parameters
callback
:函数。The callback to perform when a chat pop-out window is opened.
Example
<scripttype="text/javascript">
zE('webWidget:on','chat:popout',function(){
console.log('Live chat widget has been opened in another window.');
});
script>
chat:addTags
zE('webWidget', 'chat:addTags', tags
Add tags to a given chat session to provide extra context.
Parameters
tags
: Array. Tags to add to a given chat session. Note that the API also supports providing tags as separate string arguments (see example below).
Passing non-string types results in an error. No message is sent.
Example
<scripttype="text/javascript">
zE('webWidget','chat:addTags',['help_center','change_password']);
zE('webWidget','chat:addTags','help_center','change_password');// separate string arguments
script>
chat:removeTags
zE('webWidget', 'chat:removeTags', tags
Remove tags from a given chat session.
Parameters
tags
: Array. Tags to remove from a given chat session. Note that the API also supports providing tags as separate string arguments (see example below).
Passing non-string types results in an error. No message is sent.
Example
<scripttype="text/javascript">
zE('webWidget','chat:removeTags',['help center','change password']);
zE('webWidget','chat:removeTags','help center','change password');// separate string arguments
script>
chat:reauthenticate
zE('webWidget', 'chat:reauthenticate');
Reauthenticate users during a session. This API is particularly useful in these cases:
- Signing in a new user during an anonymous session without reloading the page.
- Signing in a new user user after a previous user has logged out without reloading the page.
Note: The API only applies authentication if thejwtFn
is provided. Please seeauthenticatesettings for more details.
Example
<scripttype="text/javascript">
zE('webWidget','chat:reauthenticate');
script>